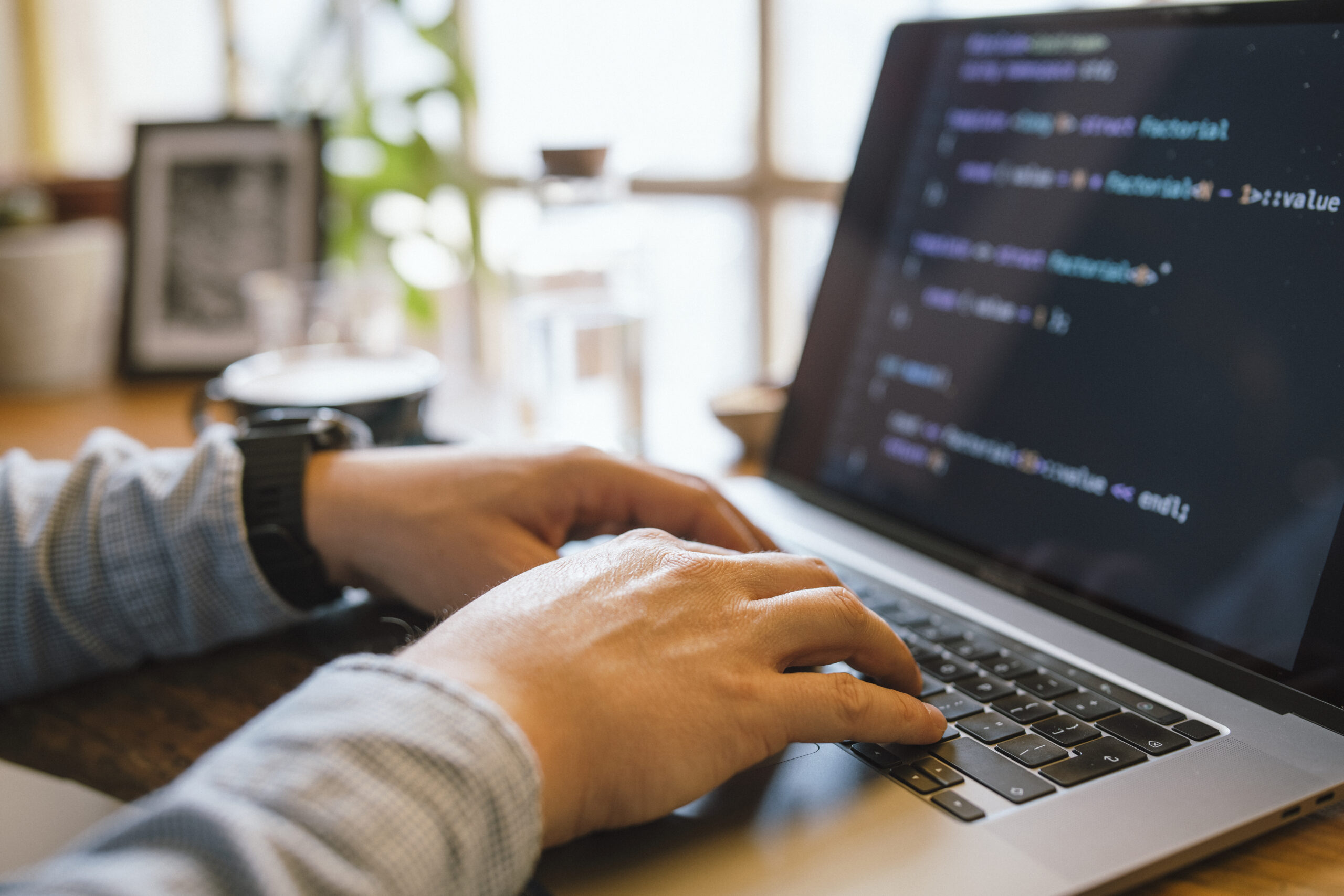
Debugging is One of the more important — nevertheless normally overlooked — skills inside a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why items go Mistaken, and Mastering to Assume methodically to unravel challenges competently. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can preserve hrs of annoyance and considerably transform your efficiency. Here's many approaches to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
Among the quickest techniques developers can elevate their debugging skills is by mastering the applications they use on a daily basis. When writing code is a person Component of growth, realizing how you can connect with it properly through execution is equally important. Modern-day advancement environments arrive Geared up with highly effective debugging capabilities — but many builders only scratch the surface of what these applications can do.
Consider, for example, an Built-in Growth Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools permit you to established breakpoints, inspect the value of variables at runtime, move via code line by line, and perhaps modify code about the fly. When employed correctly, they Enable you to observe particularly how your code behaves all through execution, that's invaluable for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-conclusion developers. They help you inspect the DOM, keep track of community requests, view true-time overall performance metrics, and debug JavaScript in the browser. Mastering the console, resources, and community tabs can change disheartening UI concerns into workable jobs.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate more than running processes and memory administration. Discovering these equipment could possibly have a steeper learning curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be comfy with Model Command methods like Git to be familiar with code history, locate the precise minute bugs ended up released, and isolate problematic changes.
In the long run, mastering your applications means going beyond default settings and shortcuts — it’s about building an personal expertise in your development atmosphere in order that when concerns occur, you’re not missing at midnight. The higher you understand your equipment, the more time you'll be able to devote fixing the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the trouble
Just about the most vital — and infrequently neglected — methods in successful debugging is reproducing the trouble. Just before jumping into your code or creating guesses, developers have to have to produce a regular surroundings or scenario where the bug reliably appears. Without the need of reproducibility, correcting a bug turns into a sport of chance, normally bringing about squandered time and fragile code adjustments.
The first step in reproducing a dilemma is collecting as much context as is possible. Question inquiries like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater depth you've, the a lot easier it gets to isolate the precise problems below which the bug occurs.
When you finally’ve collected plenty of details, try to recreate the challenge in your neighborhood ecosystem. This might necessarily mean inputting precisely the same data, simulating related user interactions, or mimicking technique states. If The difficulty seems intermittently, consider composing automatic exams that replicate the sting cases or condition transitions involved. These assessments don't just aid expose the condition but additionally protect against regressions in the future.
In some cases, The problem can be atmosphere-distinct — it'd happen only on specific running techniques, browsers, or underneath particular configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms might be instrumental in replicating these types of bugs.
Reproducing the problem isn’t only a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical tactic. But as you can consistently recreate the bug, you're presently halfway to repairing it. Using a reproducible state of affairs, you can use your debugging tools much more efficiently, examination prospective fixes securely, and talk extra Evidently with all your workforce or buyers. It turns an summary criticism right into a concrete problem — Which’s the place developers thrive.
Study and Comprehend the Error Messages
Mistake messages tend to be the most precious clues a developer has when some thing goes Incorrect. Instead of seeing them as disheartening interruptions, builders need to master to take care of error messages as direct communications from the procedure. They generally inform you what exactly took place, the place it happened, and sometimes even why it transpired — if you understand how to interpret them.
Commence by studying the information carefully As well as in entire. Numerous builders, particularly when under time tension, glance at the initial line and instantly start building assumptions. But deeper in the mistake stack or logs might lie the legitimate root trigger. Don’t just duplicate and paste error messages into search engines like google — browse and recognize them initial.
Crack the error down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line quantity? What module or function induced it? These questions can information your investigation and point you toward the liable code.
It’s also beneficial to understand the terminology in the programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Finding out to acknowledge these can dramatically speed up your debugging process.
Some problems are imprecise or generic, As well as in These scenarios, it’s crucial to examine the context through which the error transpired. Test related log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These often precede greater difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Discovering to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a a lot more successful and self-confident developer.
Use Logging Sensibly
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When made use of correctly, it offers serious-time insights into how an software behaves, encouraging you realize what’s taking place beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging method begins with understanding what to log and at what stage. Frequent logging amounts contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic data during enhancement, Facts for normal functions (like profitable commence-ups), WARN for opportunity issues that don’t crack the appliance, ERROR for actual complications, and Deadly once the system can’t go on.
Prevent flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure essential messages and decelerate your technique. Give attention to key gatherings, condition adjustments, input/output values, and significant choice details with your code.
Format your log messages Plainly and regularly. Involve context, which include timestamps, ask for IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all devoid of halting the program. They’re Specially valuable in creation environments where stepping by way of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. By using a well-considered-out logging approach, it is possible to lessen the time it will take to spot difficulties, gain deeper visibility into your apps, and Increase the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a technical job—it's a sort of investigation. To correctly discover and take care of bugs, builders should strategy the method similar to a detective resolving a mystery. This state of mind aids stop working complex problems into manageable elements and comply with clues logically to uncover the foundation induce.
Get started by gathering evidence. Consider the indicators of the situation: mistake messages, incorrect output, or general performance challenges. Just like a detective surveys against the law scene, collect just as much applicable details as you'll be able to without having jumping to conclusions. Use logs, check instances, and user reports to piece together a transparent photograph of what’s happening.
Next, variety hypotheses. Talk to you: What can be resulting in this habits? Have any variations just lately been manufactured towards the codebase? Has this issue happened in advance of underneath equivalent situations? The goal should be to slender down opportunities and establish likely culprits.
Then, check your theories systematically. Try to recreate the condition in a very controlled atmosphere. For those who suspect a certain perform or ingredient, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the effects direct you nearer to the truth.
Pay near awareness to tiny details. Bugs generally conceal during the minimum envisioned areas—similar to a missing semicolon, an off-by-one mistake, or even a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without the need of completely being familiar with it. Short term fixes may conceal the real dilemma, just for it to resurface later.
And lastly, keep notes on That which you tried and realized. Equally as detectives log their investigations, documenting your debugging procedure can preserve time for potential difficulties and assist Some others understand your reasoning.
By contemplating similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be more practical at uncovering concealed problems in intricate units.
Write Exams
Composing assessments is among the simplest methods to boost your debugging skills and General growth effectiveness. Assessments don't just help catch bugs early but additionally serve as a safety Internet that provides you confidence when creating adjustments to the codebase. A very well-analyzed software is much easier to debug mainly because it allows you to pinpoint exactly exactly where and when an issue happens.
Begin with unit exams, which give attention to specific features or modules. These modest, isolated assessments can promptly expose no matter if a selected piece of logic is working as envisioned. Any time a exam fails, you promptly know where by to glance, appreciably cutting down enough time invested debugging. Unit tests are especially useful for catching regression bugs—challenges that reappear immediately after Earlier getting set.
Next, combine integration exams and stop-to-end tests into your workflow. These help make sure a variety of elements of your application get the job done collectively smoothly. They’re significantly valuable for catching bugs that take place in complex devices with many elements or solutions interacting. If a little something breaks, your assessments can tell you which Component of the pipeline failed and less than what problems.
Writing assessments also forces you to Assume critically regarding your code. To test a attribute effectively, you would like to grasp its inputs, envisioned outputs, and edge scenarios. This degree of knowledge By natural means potential customers to better code framework and less bugs.
When debugging a difficulty, writing a failing take a look at that reproduces the bug can be a strong starting point. Once the examination fails consistently, it is possible to focus on fixing the bug and enjoy your test go when The difficulty is settled. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from the discouraging guessing game into a structured and predictable approach—helping you catch a lot more bugs, speedier plus more reliably.
Consider Breaks
When debugging a tricky situation, it’s uncomplicated to be immersed in the problem—staring at your screen for hours, making an attempt Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new standpoint.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code that you choose to wrote just several hours before. In this particular condition, your Mind gets considerably less productive at difficulty-solving. A brief wander, a espresso break, or perhaps switching to a different endeavor for ten–15 minutes can refresh your target. A lot of developers report discovering the foundation of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist prevent burnout, In particular for the duration of for a longer time debugging sessions. Sitting before a display, mentally trapped, is not merely unproductive but additionally draining. Stepping absent means that you can return with renewed Vitality and a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
When you’re stuck, a very good guideline is to set a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all over, stretch, or do some thing unrelated to code. It could really feel counterintuitive, In more info particular below restricted deadlines, however it essentially leads to more rapidly and more effective debugging Over time.
To put it briefly, using breaks will not be a sign of weak point—it’s a wise method. It presents your brain Area to breathe, enhances your standpoint, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Each and every Bug
Each individual bug you experience is much more than simply A short lived setback—it's an opportunity to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each one can teach you one thing worthwhile for those who take some time to mirror and assess what went Erroneous.
Start by asking yourself several crucial inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with better practices like unit tests, code reviews, or logging? The answers usually reveal blind spots inside your workflow or comprehending and enable you to Construct more powerful coding routines shifting forward.
Documenting bugs can also be an excellent pattern. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or popular faults—you can proactively keep away from.
In group environments, sharing Everything you've learned from the bug using your peers can be Primarily highly effective. Regardless of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll get started appreciating them as crucial parts of your growth journey. In the end, a lot of the greatest builders usually are not those who compose fantastic code, but people who consistently find out from their issues.
Ultimately, Each individual bug you repair provides a new layer to the talent established. So future time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging techniques takes time, follow, and endurance — but the payoff is huge. It would make you a far more efficient, assured, and able developer. The next time you are knee-deep in a very mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.